tl;dr : This is not a post for mining bitcoin using the RingCentral SMS app, I intend to use their SMS API to get a notification when a certain cryptocurrency changes price.
I decided to start learning about new cryptocurrencies and invest in digital markets. There is a particular new crypto called Bitcoin Vault that caught my attention.
It is a new and completely decentralized cryptocurrency that is part of the Decentralized Digital Mining Standard with new anti-theft solutions, already available in 3 exchanges. I started mining it at mininigcity.com, if you want to learn more please contact me or use my referral code: https://me.miningcity.com/referral-register/datagum
I want to keep a constant eye on its current price, so I want to be informed when the price changes. I decided to create a script that will send me a SMS using Ring Central’s API whenever the current price varies more than $10 usd (up or down) comparing it with the last price checked.
Ingredients needed :
- Ring Central Dev Account: https://developers.ringcentral.com/
- PHP : https://www.php.net/
- CoinMarketCap API: https://coinmarketcap.com/api
- Cron Job
Steps:
1.- Create a CoinMarketCap API
I use coinmarketcap to check the price of a cryptocurrency, so head to https://coinmarketcap.com/api/ and create a new developer free account.
2.- PHP Script
For this test, I created a folder named RingCentral, and added a php file named cryptoCurrency.php
Open your empty file and copy these lines of code:
<?php
$url = 'https://pro-api.coinmarketcap.com/v1/cryptocurrency/map';
$parameters = [
'symbol' => 'BTC,BTCV'
];
$headers = [
'Accepts: application/json',
'X-CMC_PRO_API_KEY: b54bcf4d-1bca-4e8e-9a24-22ff2c3d462c'
];
$qs = http_build_query($parameters); // query string encode the parameters
$request = "{$url}?{$qs}"; // create the request URL
$curl = curl_init(); // Get cURL resource
// Set cURL options
curl_setopt_array($curl, array(
CURLOPT_URL => $request, // set the request URL
CURLOPT_HTTPHEADER => $headers, // set the headers
CURLOPT_RETURNTRANSFER => 1 // ask for raw response instead of bool
));
$response = curl_exec($curl); // Send the request, save the response
print_r(json_decode($response)); // print json decoded response
curl_close($curl); // Close request
?>
In this example we are using the /map
endpoint.
Make sure to use your own API KEY or it won’t work, run the script, and you will fetch all data related to the symbols sent in the payload parameters: 'symbol' => 'BTC,BTCV'
In this example we can see data for the Bitcoin and Bitcoin Vault currency. This is useful because the API recommends using the internal id instead of the symbol or name.
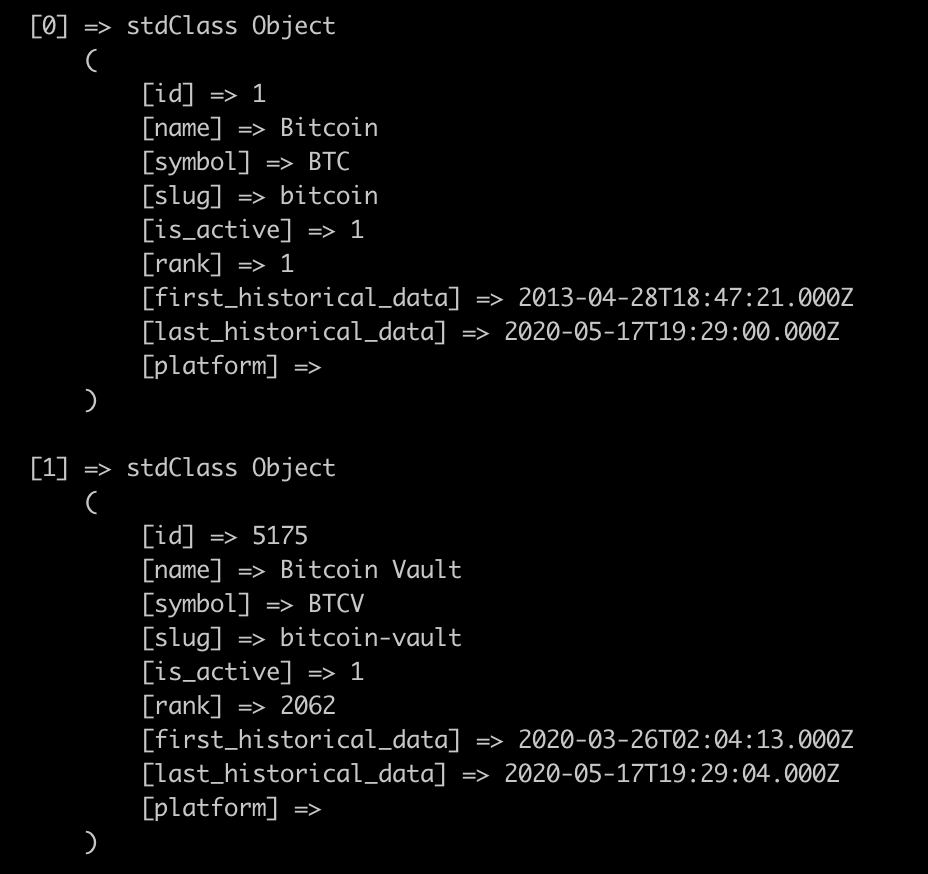
Now that we have the id (5175) for Bitcoin Vault, let’s use the endpoint that will fetch the current price: /v1/cryptocurrency/quotes/latest
Copy the following script:
<?php
$url = 'https://pro-api.coinmarketcap.com/v1/cryptocurrency/quotes/latest';
$parameters = [
'id' => '5175'
];
$headers = [
'Accepts: application/json',
'X-CMC_PRO_API_KEY: b54bcf4d-1bca-4e8e-9a24-22ff2c3d462c'
];
$qs = http_build_query($parameters); // query string encode the parameters
$request = "{$url}?{$qs}"; // create the request URL
$curl = curl_init(); // Get cURL resource
// Set cURL options
curl_setopt_array($curl, array(
CURLOPT_URL => $request, // set the request URL
CURLOPT_HTTPHEADER => $headers, // set the headers
CURLOPT_RETURNTRANSFER => 1 // ask for raw response instead of bool
));
$response = curl_exec($curl); // Send the request, save the response
$result = json_decode($response,true); // json decoded response
print_r($result['data']['5175']['quote']['USD']);
curl_close($curl); // Close request
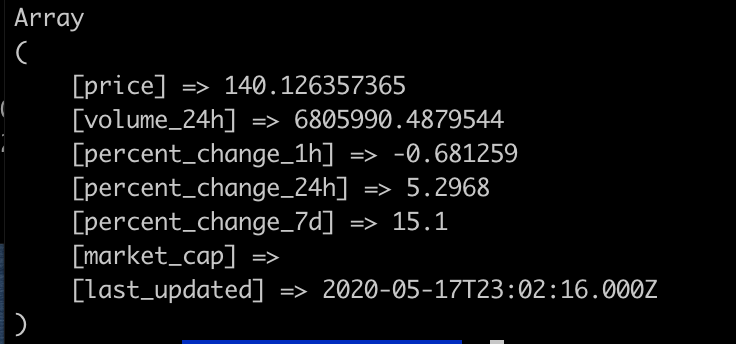
This will print the only part of the response that I am interested, the price:
In order to compare the price we need to at least have a starting point to compare, so we have to create a file where we are going to save the current price.
Create a file called currentBitcoinVaultValue.txt and add the value of 10
What we are going to do is add to our script a code that will read the current value in the file, and then update it with the current bitcoin vault price read from the API.
Copy the following script that will read the current price of BitcoinVault and save it to the .txt file:
<?php
$url = 'https://pro-api.coinmarketcap.com/v1/cryptocurrency/quotes/latest';
$parameters = [
'id' => '5175'
];
$headers = [
'Accepts: application/json',
'X-CMC_PRO_API_KEY: b5f708a1-3c63-4152-9e37-3a4a883e3820'
];
$qs = http_build_query($parameters); // query string encode the parameters
$request = "{$url}?{$qs}"; // create the request URL
$curl = curl_init(); // Get cURL resource
// Set cURL options
curl_setopt_array($curl, array(
CURLOPT_URL => $request, // set the request URL
CURLOPT_HTTPHEADER => $headers, // set the headers
CURLOPT_RETURNTRANSFER => 1 // ask for raw response instead of bool
));
$response = curl_exec($curl); // Send the request, save the response
$result = json_decode($response,true); // json decoded response
$currentBitcoinVaultPrice = $result['data']['5175']['quote']['USD']['price'];
curl_close($curl); // Close request*/
$fileName = 'currentBitcoinVaultValue.txt';
$processFile = fopen($fileName, "r") or die("Unable to open file!");
$oldPrice = fgets($processFile);
file_put_contents($fileName, $currentBitcoinVaultPrice);
My current .txt file now has been updated with the current BitcoinVault price
3.- Add Ring Central’s SMS API to our script
Now let’s continue with the fun part, get an alert when the price has changed. For this tutorial, I want to know when the prices has gone up 10 usd up or 10 usd down. So let’s add the Ring Central SMS API first.
Again I won’t go into details on how to setup an account with Ring Central, you can follow step by step on this post, check step 5(Setup RingCentral SMS API) it is pretty easy and quick to get a developer account.
Let’s add RingCentral’s API, your script should look like these:
<?php
require('vendor/autoload.php');
// CoinMarketCap API
$url = 'https://pro-api.coinmarketcap.com/v1/cryptocurrency/quotes/latest';
$parameters = [
'id' => '5175'
];
$headers = [
'Accepts: application/json',
'X-CMC_PRO_API_KEY: b5f708a1-3c63-4152-9e37-3a4a883e3820
'
];
$qs = http_build_query($parameters); // query string encode the parameters
$request = "{$url}?{$qs}"; // create the request URL
$curl = curl_init(); // Get cURL resource
// Set cURL options
curl_setopt_array($curl, array(
CURLOPT_URL => $request, // set the request URL
CURLOPT_HTTPHEADER => $headers, // set the headers
CURLOPT_RETURNTRANSFER => 1 // ask for raw response instead of bool
));
$response = curl_exec($curl); // Send the request, save the response
$result = json_decode($response,true); // json decoded response
$currentBitcoinVaultPrice = $result['data']['5175']['quote']['USD']['price'];
curl_close($curl); // Close request*/
$fileName = 'currentBitcoinVaultValue.txt';
$processFile = fopen($fileName, "r") or die("Unable to open file!");
$oldPrice = fgets($processFile);
file_put_contents($fileName, $currentBitcoinVaultPrice);
$calc = (float)$currentBitcoinVaultPrice - (float)$oldPrice;
if ($calc >= 10)
{
smsRingCentralAlert('Bitcoin Vault has gone up to '.$currentBitcoinVaultPrice);
}
if($calc <= -10)
{
smsRingCentralAlert('Bitcoin Vault has gone down to '.$currentBitcoinVaultPrice);
}
// Call Ring Central SMS API
function smsRingCentralAlert($message)
{
$RECIPIENT = 'YOUR-TEST-PHONE-NUMBER';
$RINGCENTRAL_CLIENTID = 'YOUR-
CLIENT-ID';
$RINGCENTRAL_CLIENTSECRET = 'YOUR-SECRE
T';
$RINGCENTRAL_SERVER = 'https://platform.devtest.ringcentral.com';
$RINGCENTRAL_USERNAME = 'YOUR-USERNAME';
$RINGCENTRAL_PASSWORD = 'YOUR-
PASSWORD';
$RINGCENTRAL_EXTENSION = 'YOUR-EXTENSION';
$rcsdk = new RingCentral\SDK\SDK($RINGCENTRAL_CLIENTID, $RINGCENTRAL_CLIENTSECRET, $RINGCENTRAL_SERVER);
$platform = $rcsdk->platform();
$platform->login($RINGCENTRAL_USERNAME, $RINGCENTRAL_EXTENSION, $RINGCENTRAL_PASSWORD);
$platform->post('/account/~/extension/~/sms',
[
'from' => ['phoneNumber' => $RINGCENTRAL_USERNAME],
'to' => [['phoneNumber' => $RECIPIENT]],
'text' => $message
]
);
}
Remember to you use your own API keys.
I made a couple examples using a lower and an greater price to test the script, here are my test results:
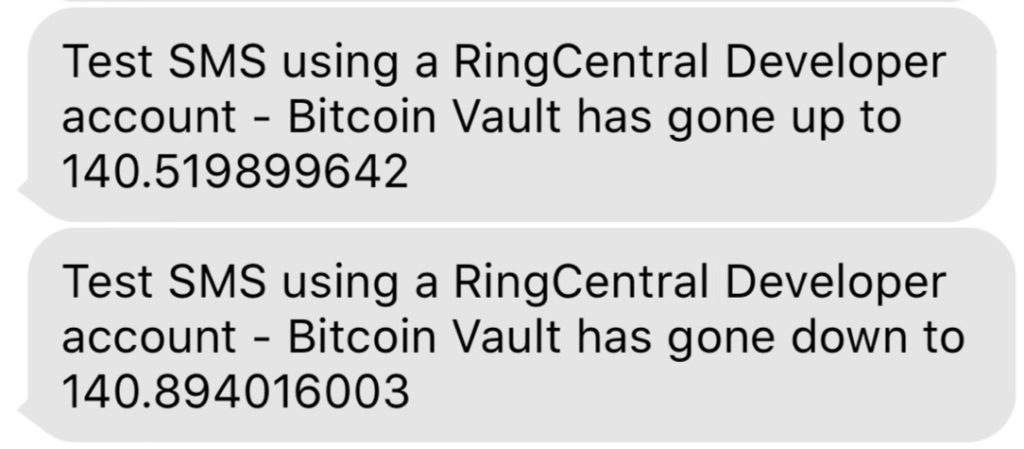
4.- Final step Cron Job
In the last tutorial I explained what a cron job is, and how to set it up, you can take a look at all the details here:
For this example I will create a cron job that will trigger our script every hour.
To create a cron job just open your CLI and type:
crontab -e
Make sure to check your current path to your PHP exe. This command will help:
whereis php
The php path is usually :/usr/bin/php
After you run the crontab, most likely an editor will open, this is an example of the cron I used for this example that will run every hour:0 */1 * * *. /usr/bin/php /Users/juan/DEV/RingCentral/cryptoCurrency.php >/tmp/stdout.log 2>/tmp/stderr.log
You can change the timing for the cron job, and also change the id for the currency that you want to check the price for.